luceos Thanks for your reply. 💗
After I register type , the alert and email is disable now. I think it's because I override it. But I don't know where I override it.
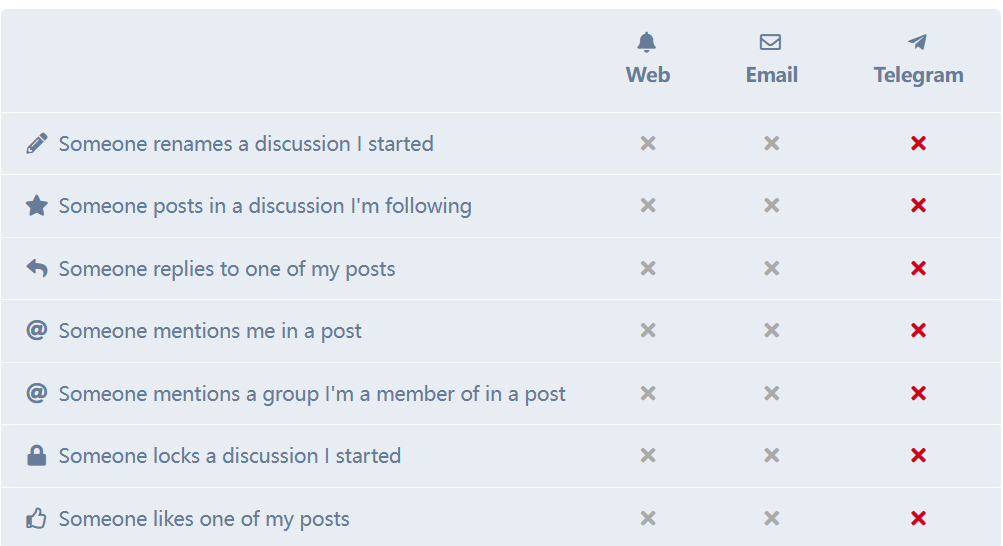
Here is my TelegramNotificationDriver code
<?php
namespace Nodeloc\Telegram\Driver;
use Flarum\Notification\Driver\NotificationDriverInterface;
use Flarum\User\User;
use Illuminate\Contracts\Queue\Queue;
use Nodeloc\Telegram\Notifications\TelegramMailer;
use Flarum\Notification\Blueprint\BlueprintInterface;
class TelegramNotificationDriver implements NotificationDriverInterface
{
/**
* @var Queue
*/
protected $queue;
public function __construct(Queue $queue)
{
$this->queue = $queue;
}
public function subscribe($events)
{
$events->listen(Sending::class, [$this, 'send']);
}
/**
* {@inheritDoc}
* @throws \Exception
*/
public function send(BlueprintInterface $blueprint, array $users): void
{
if (count($users)) {
$this->queue->push(new TelegramMailer($blueprint, $users));
}
}
protected function shouldSendTelegramToUser($blueprint, $user)
{
if (!$user->getPreference(User::getNotificationPreferenceKey($blueprint::getType(), 'telegram'))) {
return false;
}
return $this->getTelegramId($user);
}
protected function getTelegramId($actor)
{
$provider = $actor->LoginProviders()->where('provider', '=', 'telegram')->first();
return $provider->identifier;
}
public function registerType(string $blueprintClass, array $driversEnabledByDefault): void
{
User::registerPreference(
User::getNotificationPreferenceKey($blueprintClass::getType(), 'telegram'),
'boolval',
in_array('telegram', $driversEnabledByDefault)
);
}
}
And here is the service provider code:
<?php
namespace Nodeloc\Telegram\Provider;
use Flarum\Foundation\AbstractServiceProvider;
use Nodeloc\Telegram\Driver\TelegramNotificationDriver;
class TelegramNotificationServiceProvider extends AbstractServiceProvider
{
/**
* {@inheritdoc}
*/
public function register()
{
$this->container->extend('flarum.notification.drivers', function () {
return [
'telegram' => TelegramNotificationDriver::class];
});
}
}
Here is my extend.php code
<?php
namespace Nodeloc\Telegram;
use Flarum\Api\Serializer\UserSerializer;
use Flarum\Extend;
use Nodeloc\Telegram\Controllers\TelegramAuthController;
use Nodeloc\Telegram\Listeners\AddUserAttributes;
use Nodeloc\Telegram\Provider\TelegramNotificationServiceProvider;
return [
(new Extend\Frontend('forum'))
->js(__DIR__.'/js/dist/forum.js')
->css(__DIR__.'/resources/less/forum.less'),
(new Extend\Locales(__DIR__ . '/resources/locale')),
(new Extend\Event)
->subscribe(Driver\TelegramNotificationDriver::class)
->subscribe(Listeners\EnableTelegramNotifications::class)
->subscribe(Listeners\InjectSettings::class),
(new Extend\ApiSerializer(UserSerializer::class))->attributes(AddUserAttributes::class),
(new Extend\ServiceProvider())
->register(TelegramNotificationServiceProvider::class),
(new Extend\Settings)
->serializeToForum('nodeloc-telegram.botUsername', 'nodeloc-telegram.botUsername', 'boolval')
->serializeToForum('nodeloc-telegram.botToken', 'nodeloc-telegram.botToken', 'boolval')
->serializeToForum('nodeloc-telegram.enableNotifications', 'nodeloc-telegram.enableNotifications', 'boolval'),
(new Extend\Frontend('admin'))
->js(__DIR__.'/js/dist/admin.js'),
(new Extend\Routes('forum'))
->get('/auth/telegram', 'auth.telegram', TelegramAuthController::class),
];